- #include<bits/stdc++.h>
- using namespace std;
- #define ll long long int
- void computeLPSArray(string pattern, int m, int lps[]){
- int len=0;
- lps[0]=0; //lps[0] is always 0
- int i=1;
- while(i<m){
- if(pattern[i]==pattern[len]){
- len++;
- lps[i]=len;
- i++;
- }else{
- if(len!=0)
- len=lps[len-1];
- else{
- lps[i]=0;
- i++;
- }
- }
- }
- }
- void kmpSearch(string str,string pattern){
- int m=pattern.length();
- int n=str.length();
- int lps[m]; //longest prefix suffix array
- computeLPSArray(pattern,m,lps);
- int i=0; //text
- int j=0; //pattern
- bool found=0;
- while(i<n){
- if(pattern[j]==str[i]){
- i++;
- j++;
- }
- if(j==m){
- cout<<"Pattern found at index "<<i-j<<endl;
- j=lps[j-1];
- found=1;
- }else if(i<n && pattern[j]!=str[i]){
- if(j!=0) j=lps[j-1];
- else i=i+1;
- }
- }
- if(!found)cout<<"Pattern Not Found"<<endl;
- }
- int main(){
- //freopen("input.txt","r",stdin);
- //freopen("output.txt","w",stdout);
- int t;
- cin>>t;
- while(t--){
- string str,pattern;
- cin>>str>>pattern;
- kmpSearch(str,pattern);
- }
- return 0;
- }
KMP - Knuth-Morris-Pratt Pattern Matching Basic
Labels:
KMP
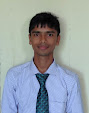
[ LightOJ ] 1255- Substring Frequency
Author : Anwar Jahid Ruman Hossain CSE, Batch - 6 BRUR. Problem Statement : [ LightOJ ] 1255- Substring Frequency Source : URI Online Judge Category : Pattern Searching Algorithm : Z-algorithm Verdict : Accepted
- /*__//\\//\\//\\//\\//\\= BISMILLAHIR RAHMANIR RAHIM =//\\//\\//\\//\\//\\//__*/
- #include <bits/stdc++.h>
- //........................</HEADER>................................
- #include <ext/pb_ds/assoc_container.hpp> // Common file
- #include <ext/pb_ds/tree_policy.hpp> // Including tree_order_statistics_node_update
- #include <ext/pb_ds/detail/standard_policies.hpp>
- using namespace std;
- //........................</CONSTANT DEFINE>........................
- const unsigned int MOD = 1000000007;
- #define ll long long
- #define Imax 1e9+5
- #define LLmax 1e18
- #define Imin -1e9-5
- #define LLmin -1e18
- #define PI acos(-1.0)
- #define EPS 1e-9
- #define INF 1<<28
- //........................</LOOP >.................................
- #define rep(i,a,n) for(int i=a;i<n;i++)
- #define per(i,a,n) for(int i=n-1;i>=a;i--)
- //................</ STANDARTD TEMPLATE LIBRARY>...................
- #define all(x) (x).begin(),(x).end()
- #define pb(x) push_back(x)
- #define mp(a,b) make_pair((a),(b))
- //........................</ TYPE CONVERSION>......................
- #define toRad(a) ((a)*(PI)/180)
- #define toDeg(a) ((a)*180/(PI))
- //........................</ MATH>.................................
- #define max3(a,b,c) max(a,max(b,c))
- #define min3(a,b,c) min(a,min(b,c))
- #define sq(a) ((a)*(a))
- inline bool isEq(double a,double b){
- return (abs(a-b)<EPS);
- }
- template<typename T> T gcd(T a, T b){return(b?__gcd(a,b):a);}
- template<typename T> T lcm(T a, T b){return(a*(b/gcd(a,b)));}
- //........................</ ARRAY DEFAULT>........................
- #define mem(a,v) memset(a,v,sizeof(a))
- string day [] = {"SUN", "MON", "TUE", "WED", "THU", "FRI", "SAT"};
- int month[]={31,28,31,30,31,30,31,31,30,31,30,31}; //Not Leap Year
- //........................</DIRECTION>.............................
- int dx4[]={1,0,-1,0};
- int dy4[]={0,1,0,-1}; //4 Direction
- int dx8[]={1,1,0,-1,-1,-1,0,1};
- int dy8[]={0,1,1,1,0,-1,-1,-1};//8 direction
- int dxh[]={2,1,-1,-2,-2,-1,1,2};
- int dyh[]={1,2,2,1,-1,-2,-2,-1};//Knight Direction (horse)
- //........................</ INPUT/OUTPUT>.........................
- #define read(n) scanf("%d",&n)
- #define write(n) printf("%d",n)
- #define endl '\n'
- #define PRECISION(x) cout << fixed << setprecision(x)
- #define FAST_IO ios::sync_with_stdio(0); cin.tie(0); cout.tie(0);
- //.......................</ PRE DEFINED FUNCTIONS>..................
- bool sortByFirst(const pair<int,int>&a,const pair<int,int>&b){
- return a.first>b.first;
- }
- //vector <string> v;
- //void dfs (string str, string t = "", int cnt = 0) {
- // if (cnt == 2) {
- // v.push_back (t);
- // return;
- // } else {
- // for (int i = 0; i <(int)str.size (); i ++) {
- // dfs (str, t + str [i], cnt + 1);
- // }
- // }
- //}
- //vector<vector<int> >divisor(100005);
- //void divisors(){
- // for(int i=1;i<100005;i++){
- // for(int j=i;j<100005;j+=i){
- // divisor[j].push_back(i);
- // }
- // }
- //}
- void getZarray(string str, int z[]){
- int n=str.length();
- int left,right,k;
- left=right=0;
- for(int i=1;i<n;i++){
- if(i>right){
- left=right=i;
- while(right<n && str[right-left]==str[right]){
- right++;
- }
- z[i]=right-left;
- right--;
- }else{
- k=i-left;
- if(z[k]<right-i+1){
- z[i]=z[k];
- }else{
- left=i;
- while(right<n && str[right-left]==str[right]){
- right++;
- }
- z[i]=right-left;
- right--;
- }
- }
- }
- }
- int period_count(string text,string pattern){
- string concat=pattern+"$"+text;
- int cnt=0;
- int len=concat.length();
- int z[len];
- getZarray(concat,z);
- int flag=0;
- for(int i=1;i<len;i++){
- if(z[i]==pattern.length()){
- cnt++;
- }
- }
- return cnt;
- }
- int main () {
- FAST_IO
- // freopen("input.txt","r",stdin);
- // freopen("output.txt","w",stdout);
- int t;
- cin>>t;
- for(int i=1;i<=t;i++){
- string text,pattern;
- cin>>text>>pattern;
- cout<<"Case "<<i<<": "<<period_count(text,pattern)<<endl;
- }
- return 0;
- }
Labels:
Z-Algorithm
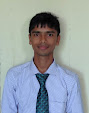
Subscribe to:
Posts (Atom)